Golang Interview Questions and Answers
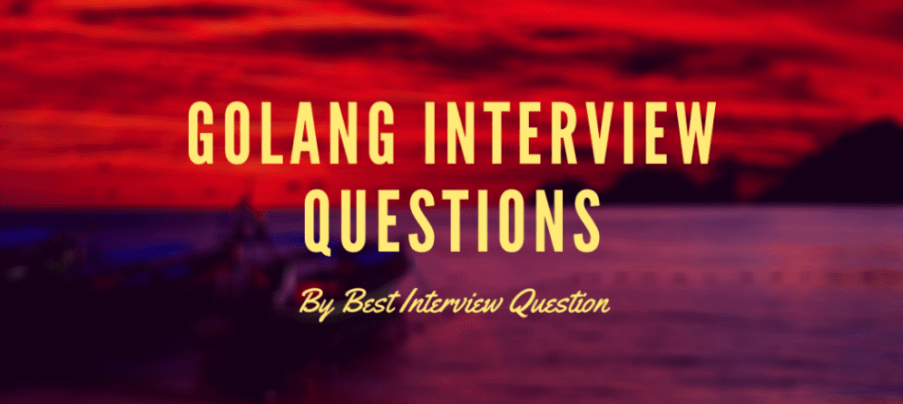
An open-source programming language developed by Google, Go is designed for building fast and reliable applications. It is a statically-typed language that has a similar syntax to C. This information has been asked quite a few times recently in Golang interview questions. Some of its main features include dynamic typing, rich library, a documentation engine called GoDoc that is used by the entire Go community, static code analysis, and built-in testing tools that are simple and efficient.
Quick Facts About Go Programming | |
---|---|
What is the latest version of Go? | 1.14.4 released on 1st June 2020. |
When did Go programming release? | 10th November 2009. |
Who is the developer of Golang? | It is designed at Google and developed by Robert Griesemer, Rob Pike, and Ken Thompson. |
What language does Golang use? | The former was written in C but now written in Go itself. |
Most Frequently Asked Golang Interview Questions
Generally, large programs are made of many multiple small subprograms. For example, a server handles multiple requests made via web browsers and serves HTML web pages in response. In this case, each request made is considered as a small program.
Golang makes it possible to run smaller components of each of these programs simultaneously through concurrency. It has extensive support for concurrency using goroutines and channels
Golang language interfaces are different from other languages. In Golang, type implements an interface through the implementation of its methods. There are no explicit declarations or no “implements” keyword.
package main
import "fmt"
type I interface {
M()
}
type T struct {
S string
}
// This method means type T implements the interface I,
// but we don't need to explicitly declare that it does so.
func (t T) M() {
fmt.Println(t.S)
}
func main() {
var i I = T{"hello"}
i.M()
}
package main
import "fmt"
func main() {
fmt.Println(functionByBestInterviewQuestion())
}
func functionByBestInterviewQuestion() []int {
a, b := 15, 10
b, a = a, b
return []int{a, b}
}
Golang's small syntax and concurrency model make it a without a doubt speedy programming language. Golang is compiled to machine code and its compilation system is very fast. Go additionally hyperlinks all the dependency libraries into a single binary file as a consequence putting off the dependency on servers.
To check if the array is empty follow these steps:
Check with the builtin len()
function, for example, len(slice) <= 0
. If the array is empty, skip the for a loop.
r := whatever()
if len(r) > 0 {
// do what you want
}
- Concise, simple to work and Scalable
- Built-in support for other applications
- Good speed across platforms like OS X, Linux, and Windows.
- Ability to cross-compile the application to run on different devices than the ones used for development
- Automatic management of memory
It is a directory hierarchy with three directories – src, pkg and bin - at its root that contain the Go code. The "src" directory includes source files, the "pkg" directory contains objects, and the "bin" directory contains commands.
Note: This information is usually asked in golang interview questions.
In Golang, a channel is a communication object which uses goroutines to communicate with each other. Technically, it is a data transfer pipe in which data can be transferred into or read from.
It is a function that runs concurrently with other functions. If you want to stop it, you will have to pass a signal channel to the goroutine, which will push a value into when you want the function to finish.
Quit : = make (chan bool)
go func ( ) {
for {
select {
case <- quit:
return
default
// do other stuff
}
}
}()
// Do stuff
// Quit goroutine
Quit <- true
Yes, Golang supports multithreading. Moreover, its design is based on multithreading.
Advantages
- Concise, simple to work and Scalable
- Built-in support for other applications
- Good speed across platforms like OS X, Linux, and Windows.
- Ability to cross-compile the application to run on different devices than the ones used for development
- Automatic management of memory
Are you looking for Golang Interview Questions? As a developer, you would want to know the best possible Questions that you might be asked in your Job Interview.