React js interview questions and Answers
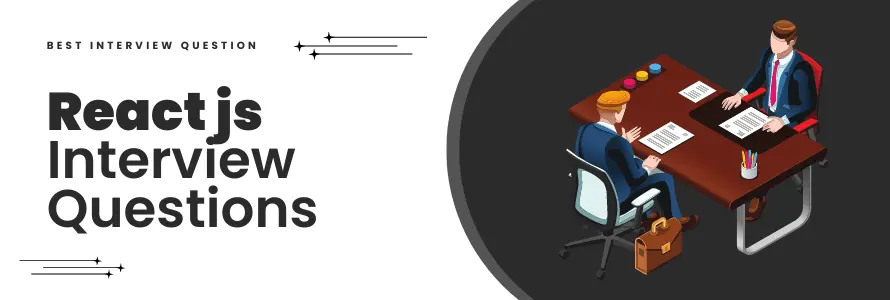
ReactJS is a JavaScript library for building reusable User interface components. It is a template-based language combined with different functions that support the HTML. Our recently updated React Interview Questions can help you crack your next interview. This means the result of any React's operation is an HTML code. You can use ReactJS to build all kinds of single-page applications.
Short Questions About React | |
---|---|
What is the latest version of React? | 18.0, released on March 29, 2022. |
When was React first released? | 29th May 2013 |
React JS is Created By | Jordan Walke |
License of ReactJS | MIT License |
React Developer Interview Questions
The term pure function in React means that the return value of the function is entirely determined by its inputs and that the execution of the function produces no side effects.
The ReactDOMServer is an object which enables you to render components as per a static markup. Generally, it is used on a Node Server.
The App.proptypes is used for validating props in React components.
Here is the syntax:
class App extends React.Component {
render() {}
}
Component.propTypes = { /*Definition */};
There are 3 ways to create a component in React:
- Using a depreciated variable function
- Using a Class
- Using a Stateless Functional Component
1. Using a depreciated variable function
In this, you need to declare a variable in your Javascript file/tag and use the React.createClass() function to create a component using this code.
var MyComponent = React.createClass({
render() {
return <div>
<h1>Hello World!</h1>
<p>This is my first React Component.</p>
</div>
}
})
Now, use the div element to create a unique ID for the specific page and then link the above script onto the HTML page.
<div id=”react-component”></div>
Use ReactDOM.render()
function to take 2 arguments, the react component variable and the targeted HTML page.
ReactDOM.render(<MyComponent />, document.getElementById('react-component'))
Finally, save the HTML page to display the result like this one below:
2. Using a Class
Javascript has lately started supporting classes. Any React developer can easily create a class using the class extends (Inherits). Following is the code
3. Using a Stateless Functional Component
It might sound a bit complex, but this basically means that a normal function will be used in place of a variable to return a react component.
Create a const called MyComp and set it as equal to a () function. Use the arrow function, => to declare the logic of the function as follows:
const MyComponent = () => {
return <div>
<h1>Hello!</h1>
<p>This is my first React Component.</p>
</div>
}
There are two ways to link one page to another to React:
Using React Router: Use the feature from the react-router to link the first page to the second one.
<Link to ='/href' ></Link>
Reloading/Refreshing a page using Javascript:
Use Vanilla JS to call the reload method for the specific page using the following code:
window.location.reload(false);
The best way to redirect a page in React.js depends on what is your use case. Let us get a bit in detail:
If you want to protect your route from unauthorized access, use the
import React from 'react'
import { Redirect } from 'react-router-dom'
const ProtectedComponent = () => {
if (authFails)
return <Redirect to='/login' />
}
return <div> My Protected Component </div>
}
In React, an object this.refs which is found as an instance inside the component can be used to access DOM elements in Reactjs.
Note: One of the most tricky react advanced interview questions, you need to answer this question with utmost clarity. Maybe explaining it with a short example or even a dry run would help you get that dream job.
React Hooks were brought in by the React team to efficiently handle state management and the side-effects in function components. React Hooks is a way of making the coding effortless. They help by enabling us to write React applications by only using React components.
To render a component with another component in Reactjs, let us take an example:
Let us suppose there are 2 components as follows:
Component 1
class Header extends React.Component{
constructor(){
super();
}
checkClick(e, notyId){
alert(notyId);
}
}
export default Header;
Component 2
class PopupOver extends React.Component{
constructor(){
super();
}
render(){
return (
<div className="displayinline col-md-12 ">
Hello
</div>
);
}
}
export default PopupOver;
Now to call the Component 1 in Component 2, use this code:
import React from 'react';
class Header extends React.Component {
constructor() {
super();
}
checkClick(e, notyId) {
alert(notyId);
}
render() {
return (
<PopupOver func ={this.checkClick } />
)
}
};
class PopupOver extends React.Component {
constructor(props) {
super(props);
this.props.func(this, 1234);
}
render() {
return (
<div className="displayinline col-md-12 ">
Hello
</div>
);
}
}
export default Header;
Top 20 React.js Interview Questions
Here you will find the list of Top React interview questions, which were written under the supervision of industry experts. These are the most common questions and usually asked in every interview for the role of the React Developers.
- What is HOC?
- What are the difference between Rest and Spread Operator?
- What are the key benefits of using Arrow functions?
- What is Pure Component?
- What are Controlled and Uncontrolled components with example?
- What is Refs?
- What is the difference between class based component & function based components?
- How to work React?
- Why virtual Dom is faster than real dom?
- How does virtual Dom work in react?
- What are the difference between null & undefined?
Conclusion
Now that you have read all the react questions, it is time for you to go towards the next level. Applying for a developer position would require you to actually code and not just give answers to theoretical questions.
That’s why you should enhance your skills by complementing the above react developer interview questions with a practical mindset. Start coding small stuff, get your concepts crystal clear. It’s all about how you can apply your knowledge in different projects. Code and code, that’s the secret to winning the dream job!