Top React Native Interview Questions for Job Seekers
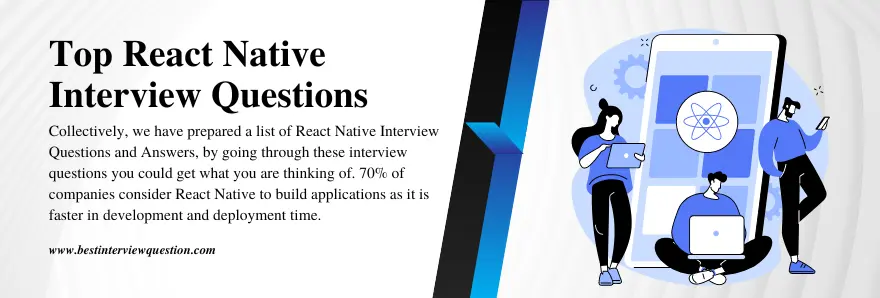
React Native is one of the most popular mobile application frameworks developed by Facebook and is based on JavaScript. This framework is growing so fast in the market that it is used by more than 8000 companies and these companies include names like Facebook, Instagram, Airbnb, etc. To be a part of these companies could be a dream for anyone and to achieve this dream you have to be a React developer. Collectively, we have prepared a list of React Native Interview Questions and Answers, by going through these interview questions you could get what you are thinking of. 70% of companies consider React Native to build applications as it is faster in development and deployment time.
Top 10 React Native Interview Questions for 2023
- What is the purpose of a Native module in React Native, and how do you create one?
- How does React Native handle layout and styling compared to CSS?
- How do you debug a React Native application?
- What is the difference between AsyncStorage and LocalStorage in React Native?
- How do you handle navigation between screens in a React Native application?
- Can you explain the concept of React Native bridge and how it works?
- What is the purpose of React Native's "Flexbox" layout, and how does it work?
- How do you handle errors in a React Native application, and what are some best practices?
- Can you explain the "Props drilling" concept in React Native and how you avoid it?
- How do you handle user authentication and authorization in a React Native application?
- Can you explain the concept of Redux-saga and how it works with Redux in React Native?
- How do you test a React Native application, and what are some best practices?
Quick Facts About React Native | |
---|---|
When did React Native Initial release? | 26th March 2015 |
What is the latest version of React Native? | React Native 0.70 was released on Sep 5, 2022 |
React Native is Created By | |
What language does React Native use? | It is based on React js, which is written in JavaScript. |
React Native Technical Interview Questions and Answers
A Native module in React Native is a way to add native code (i.e., code written in Java or Objective-C/Swift) to a React Native application. Native modules allow React Native apps to access functionality that isn't available in JavaScript, such as accessing the camera or making network requests.
To create a Native module in React Native, you typically need to perform the following steps:
- Create a new module file in your native code (either Java or Objective-C/Swift, depending on your platform) that exports the functionality you want to use in your React Native app.
- Add the React Native bridge code to your module file, which allows your JavaScript code to communicate with your native code.
- Create a new JavaScript file that defines a wrapper around your native module. This file should define a JavaScript class that provides methods that correspond to the functionality in your native module.
- Finally, add your new module to your app's package.json file, so that it can be installed and used by your app.
Here's an example of how to create a simple Native module in React Native for Android:
- Create a new Java file called MyModule.java, and add the following code:
package com.myapp;
import com.facebook.react.bridge.ReactContextBaseJavaModule;
import com.facebook.react.bridge.ReactMethod;
public class MyModule extends ReactContextBaseJavaModule {
public MyModule(ReactApplicationContext reactContext) {
super(reactContext);
}
@Override
public String getName() {
return "MyModule";
}
@ReactMethod
public void myMethod(String message) {
// Do something with the message
}
} - Add the following code to the MainApplication.java file in your Android app:
@Override
protected ListgetPackages() {
return Arrays.asList(
new MainReactPackage(),
new MyModulePackage() // <-- Add this line
);
} - Create a new JavaScript file called MyModule.js, and add the following code:
import { NativeModules } from 'react-native';
const { MyModule } = NativeModules;
export default MyModule; - Finally, add your new module to your app's package.json file, like so:
{
"name": "myapp",
"version": "0.0.1",
"private": true,
"dependencies": {
"react": "16.13.1",
"react-native": "0.63.2",
...
},
"rnpm": {
"assets": [
"assets/fonts/"
]
},
"scripts": {
"start": "react-native start",
"android": "react-native run-android",
"ios": "react-native run-ios",
"test": "jest"
},
"devDependencies": {
...
},
"react-native": {
"dependencies": {
"MyModule": {
"platforms": {
"android": null
}
}
}
}
} - After completing these steps, you should be able to use your new Native module in your React Native app by importing it from your MyModule.js file and calling its methods. For example:
import MyModule from './MyModule';
MyModule.myMethod('Hello, Native module!');
React | React Native |
---|---|
It is a JavaScript library, supporting front-end web and being run on a server, for web applications and building user interfaces. | It is a framework that compiles to native app components, allowing you to build native mobile applications for different platforms. |
React Js is a Javascript Library where you can develop and run faster web applications. | React-Native is a framework where you can develop mobile applications. |
React is for websites. | React Native is for mobile applications. |
The ReactJs is a JavaScript library to develop apps in HTML5 while using JavaScript as the developing language. Whereas, React Native is a JavaScript framework used to create native mobile applications while using JavaScript as the development language.
To install a specific version of React Native, use this following command:
$ react-native init newproject --version react-native@VersionNumber
Note: In the above, replace VersionNumber with the version of React you want to install.
The map function is used to show a list of elements in an array. It can be used in React Native like the following example:
import React, { Component } from "react";
import { Text, View } from "react-native";
export default class mapFunction extends Component {
constructor(props) {
super(props);
this.state = {
array: [
{
title: "example title 1",
subtitle: "example subtitle 1"
},
{
title: "example title 2",
subtitle: "example subtitle 2"
},
{
title: "example title 3",
subtitle: "example subtitle 3"
}
]
};
}
list = () => {
return this.state.array.map(element => {
return (
<View style={{ margin: 10 }}>
<Text>{element.title}</Text>
<Text>{element.subtitle}</Text>
</View>
);
});
};
render() {
return <View>{this.list()}</View>;
}
}
Higher-Order-Component, shortly known as HOC is an advanced React Native technique to reuse the component logic. The function obtains a component and returns a new element.
NOTE: If you are a react native developer then these questions & answers will help you to crack your interview easily.
function HOC(Comp) {
return class NewComp extends Component {
render() {
return <comp>
}
}
}
To use the camera to React Native, follow these steps carefully:
- On Android, camera permission must be asked:
<uses-permission android:name="android.permission.CAMERA" />
- Now, to enable video recording feature add the following code to the AndroidManifest.xml:
<uses-permission android:name="android.permission.RECORD_AUDIO"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
- For iOS, you must update the Info.plist with a usage description for camera
<key>NSCameraUsageDescription</key> <string>Your own description of the purpose</string>
Yes, React Native is a mobile app development framework that allows developers to build mobile applications for both iOS and Android platforms using JavaScript and React. React Native is based on React, a popular JavaScript library for building user interfaces on the web, and uses a similar approach to building mobile apps.
React Native allows developers to write code once and reuse it across multiple platforms, which can save time and resources compared to developing separate apps for each platform. The resulting apps are considered "native" because they use the same building blocks as traditional mobile apps, such as buttons, text fields, and other UI elements.
So, in summary, React Native is a mobile app development framework that enables developers to create cross-platform mobile applications using JavaScript and React.
A React component can be viewed as pure on the chance that it renders a similar output for a similar state and props. Class components that extend the React are PureComponent class that is treated as pure components.
Yes, we can combine Android or iOS code in react native. React Native helps to smoothly combines the components written in Java, Objective-C or Swift.
The use of Arrow functions in React Native is to help reduce your application's memory consumption. It is done by reducing the CPU time required to iterate the over loops to generate the components necessary for your lists.
Here’s an example of Arrow function in render:
class Foo extends Component {
handleClick() {
console.log('Click happened');
}
render() {
return <button onClick={() => this.handleClick()}>Click Me</button>;
}
}
In the end, we know an interview that has a Javascript word in it, is not very easy to crack. By going through various questions published on different websites wouldn’t open the door for you. Here are some bonus tips for you which you should also keep in mind-
- Pick a programming language and make a good command of it.
- Don’t mix concepts of ReactJS with React Native.
- Practice regularly some coding challenges and mock interviews.
- You should know different methods to solve a problem.
- Take time to think before answering, don’t hurry.