Promise in JavaScript
An object that shows the eventual completion or failure of asynchronous options along with its resultant value is called a promise in JavaScript. The possible three states of a promise are pending, fulfilled, or rejected. It allows handling multiple asynchronous operations in JavaScript.
We have a list of JavaScript Multiple Choice Questions. It's really helpful to crack your interviews easily.
A Promise has 3 states:
- Pending: initial state, neither fulfilled nor rejected.
- Fulfilled: meaning that the operation was completed successfully.
- Rejected: meaning that the operation failed.
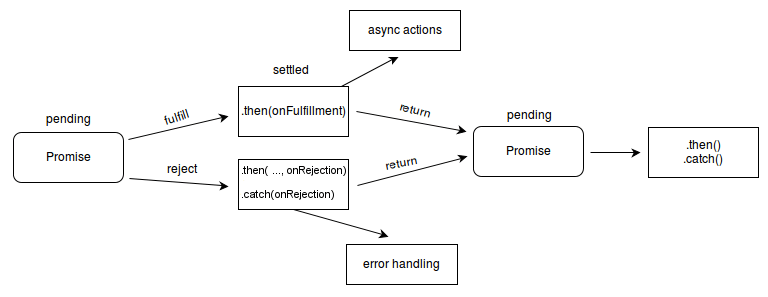
How to create Promises?
let getDataFromAPI = function(params) {
return new Promise((resolve, reject) => {
const data = {
name: "Umesh",
phone: "9971083635"
}
if(data) {
resolve(data); // get data from API
} else {
reject('get error from API Call');
}
});
}
getDataFromAPI().then( async function (result) {
console.log(result);
}).catch(err => {
console.log(err);
});
How to call multiple promise (with dependency) methods?
callApi().then(function(result) {
return callApi2() ;
}).then(function(result2) {
return callApi3();
}).then(function(result3) {
// do work
}).catch(function(error) {
//handle any error that may occur before this point
});
OR
Promise.all([callApi, callApi2, callApi3]).then((values) => {
console.log(values);
});
OR
Promise.allSettled([callApi, callApi2, callApi3]).then((values) => {
console.log(values);
});