PHP Array Functions
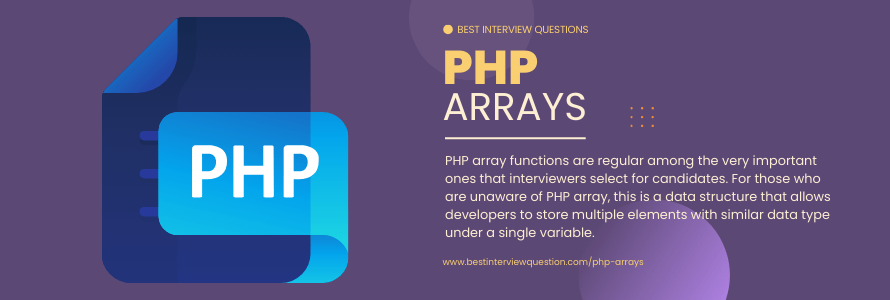
PHP array functions are regular among the very important ones that interviewers select for candidates. For those who are unaware of PHP array, this is a data structure that allows developers to store multiple elements with similar data type under a single variable, which save developers the effort to create a different variable for every data. The PHP arrays functions are extremely helpful creating a list of elements of similar types, which developers will able to access using their key or index.
Types of arrays in PHP;
- Numeric or Indexed Arrays,
- Multidimensional Arrays
- Associative Arrays
Most Frequently Asked PHP Array Functions
array_key_exists() : It is used to checks an array for a particular key and returns TRUE if the key exists and FALSE if the key does not exist.
array_keys() : This function returns an array containing the keys. It takes three parameters out of which one is mandatory and other two are optional.
Syntax : array_keys(array,value,strict)
array_merge() | array_merge_recursive() |
---|---|
This function is used to join one or more arrays into a single array. | Used to merge multiple arrays in such a manner that values of one array are appended to the end of the previous array. |
Syntax: array_merge($array1, $array2, $array3...); | Syntax: array_merge_recursive($array1, $array2, $array3...); |
If we pass a single array, it will return re-indexed as a numeric array whose index starts from zero. | If we pass a single array, it will return re-indexed in a continuous manner. |
Example of an array_merge() function:
$arrayFirst = array("Topics" => "Maths","Science", "Computers");
$arraySecond = array("Class-V", "Class-VI", "Section"=>"C");
$resultArray = array_merge($arrayFirst, $arraySecond);
print_r($resultArray);
Output
Array ( [Topics] => Maths [0] => Science [1] => Computers [2] => Class-V [3] => Class-VI [Section] => C )
Example of an array_merge_recursive() function with all different keys:
$a1=array("a"=>"Best", "b"=>"Interview");
$a2=array("z"=>"Question");
print_r(array_merge_recursive($a1, $a2));
Output
: Array
(
[a] => Best
[b] => Interview
[z] => Question
)
In PHP arrays, the array_key_exists() function is used when the user wants to check if a specific key is present inside an array. If the function returns with a TRUE value, it is present.
Here’s its syntax: array_key_exists(array_key, array_name)
Also, here is an example of how it works
$array1 = array("Orange" => 100, "Apple" => 200, "Grapes" => 300, "Cherry" => 400);
if (array_key_exists("Grapes",$array1))
{
echo "Key exists";
}
else
{
echo "Key does not exist";
}
Output:
key exists
Associative arrays are used to store key and value pairs. For example, to save the marks of the unique subjects of a scholar in an array, a numerically listed array would no longer be a nice choice.
/* Method 1 */
$student = array("key1"=>95, "key2"=>90, "key3"=>93);
/* Method 2 */
$student["key1"] = 95;
$student["key2"] = 90;
$student["key3"] = 93
In PHP, if you want to access a single value from an array, be it a numeric, indexed, multidimensional or associative array, you need to use the array index or key.
Here’s an example using a multidimensional array:
$superheroes = array(
array(
"name" => "Name",
"character" => "BestInterviewQuestion",
),
array(
"name" => "Class",
"character" => "MCA",
),
array(
"name" => "ROLL",
"character" => "32",
)
);
echo $superheroes[1]["name"];
Output:
CLASS
In PHP, pushing a value inside an array automatically creates a numeric key for itself. When you are adding a value-key pair, you actually already have the key, so, pushing a key again does not make sense. You only need to set the value of the key inside the array. Here’s an example to correctly push a [air pf value and key inside an array:
$data = array(
"name" => "Best Interview Questions"
);
array_push($data['name'], 'Best Interview Questions');
Now, here to push “cat” as a key with “wagon” as the value inside the array $data, you need to do this:
$data['name']='Best Interview Questions';
$array = array('1' => 'best', '2' => 'interview', '3' => 'question')
$array[3] = $array[5];
unset($array[3]);
// OUTPUT
array('1' => 'best', '2' => 'interview', '5' => 'question')
To select the common data from two arrays in PHP, you need to use the array_intersect() function. It compares the value of two given arrays, matches them and returns with the common values. Here’s an example:
$a1=array("a"=>"red","b"=>"green","c"=>"blue","d"=>"yellow");
$a2=array("e"=>"red","f"=>"black","g"=>"purple");
$a3=array("a"=>"red","b"=>"black","h"=>"yellow");
$result=array_intersect($a1,$a2,$a3); print_r($result);
Output:
Array ( [a] => red )
The array_keys() function is used in PHP to return with an array containing keys.
Here is the syntax for the array_keys() function: array_keys(array, value, strict)
Here is an example to demonstrate its use
$a=array("Volvo"=>"AAAAAAAA","BMW"=>"BBBBBBB","Toyota"=>"CCCCCC");
print_r(array_keys($a));
Output:
Array ( [0] => Volvo [1] => BMW [2] => Toyota )
To print all the values inside an array in PHP, use the for each loop.
Here’s an example to demonstrate it:
$colors = array("Red", "Green", "Blue", "Yellow", "Orange");
// Loop through colors array foreach($colors as $value){ echo $value . "
"; }
Output:
Red
Green
Blue
Yellow
Orange
Arrays help developers store multiple elements within a single variable that can be accessed using a key or an index. We can also combine it with for each statement to a quick loop through an array with the use of very little code. While web application development, we can store tabular data in a pair of nested arrays to achieve better performance and utility. PHP arrays functions also have various functions such as merge, sort, split and intersect which makes it easy for us to efficiently manipulate them. With the inefficiency of strong typing and OOP-object iteration support in PHP, arrays are the most preferable data structure to store and manipulate data. We will further continue here with top PHP Array interview questions picked by industry leaders with their suggested answers for your practice.