Advanced JavaScript Interview Questions
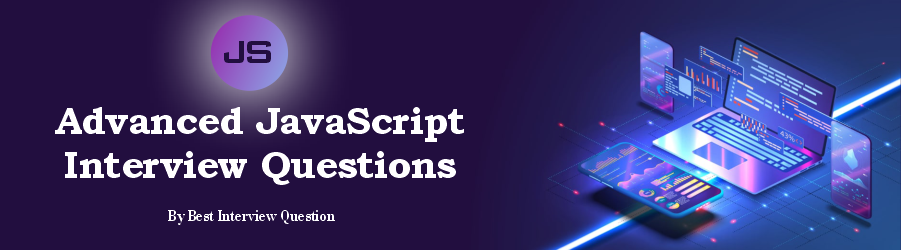
Over the years, JavaScript has become one of the most influential programming languages in the software industry. Due to this, there are a number of job applications and applicants in this industry. Due to this, we decided to come up with the actual Advanced JavaScript Interview Questions, questions that actually matter while you create codes for your company. Have a read on these questions to cement your knowledge in JavaScript and get that dream job. But, over time, we have found out that many developers lack a basic understanding of JavaScript such as working on objects and working on obsolete patterns like deeply layered inheritance hierarchies.
What's in it for me?
We have created this section for your convenience from where you can navigate to all the sections of the article. All you need to just click or tap on the desired topic or the section, and it will land you there.
JavaScript Coding Interview Questions
The primary difference between the == or === operators in JavaScript is that the == operator converts the operands before comparing, while the === operator compares both the data types and the values.
(==)
When we want to compare two values, one string, and another number, it will convert the string to the number type, then compare the results.
let a = 10;
let b = '10';
console.log(a == b); // true
(===)
let a = 10;
let b = '10';
console.log(a === b); // false
- setTimeout: It allows us to run a function after the interval of time.
- setInterval: It allows us to run a function repeatedly, starting after the interval of time, then repeating again and again continuously at that interval.
These methods are not part of JavaScript specification. These methods are provided by most environments that have an internal scheduler.
setTimeout(() => alert("JavaScript Interview Questions"), 3000); // setTimeout
setInterval(() => alert("JavaScript Interview Questions"), 3000); // setInterval
for(var x = 1; x <= 5; x++) {
setTimeout(() => console.log(x), 0);
}
6
6
6
6
6
The rest operator lets us call any function with any number of arguments and then access the excess arguments as an array. You can also use the rest operator to destroy objects or arrays.
Spread operator (...) lets you expand an iterable-like array into its individual elements.

Examples of Spread Operator
1. The following example shows two arrays that are separated by the spread operator (...). They're combined into one using this method. The elements of the merged array are in the same order as they were merged.
var array1 = [10, 20, 30, 40, 50];
var array2 = [60, 70, 80, 90, 100];
var array3 = [...array1, ...array2];
console.log(array3);
Output
[ 10, 20, 30, 40, 50, 60, 70, 80, 90, 100 ]
2. This example shows how to add an element to an iterable. A given array must be defined. We use the spread operator, which spreads all the values of iterables and adds the elements to the array in the order we choose.
const array1 = [10, 20, 30, 40, 50, 60];
const array2 = [...array1, 5, 55];
console.log(array2);
Output
[ 10, 20, 30, 40, 50, 60, 5, 55 ]
3. This example will show how to copy objects with the spread operator. Spread operator (...). gives obj2 all the properties of the original obj1. Curly brackets are required to indicate that an object is being copied, or else an error will be raised.
const array1 = [10, 20, 30, 40, 50, 60];
const array2 = [ ...array1 ];
console.log(array2);
Output
[ 10, 20, 30, 40, 50, 60 ]
The "use strict" is not a statement, rather a literal expression that is vital to your code as it presents a way for voluntarily enforcing a stricter parsing and error handling process on your JavaScript code files or functions during runtime. Most importantly, it just makes your code very easy to manage.
Here are some of the benefits of using use strict expression at the beginning of a JS source file:
- It makes debugging a lot easier by making the code errors that are ignored generate an error or throw exceptions.
- It prevents the most common error of making an undeclared variable as a global variable if it is assigned a value.
- It does not allow the usage of duplicate property names or parameter values.
Asynchronous programming means that the program engine runs in an event loop. Only when blocking operation is required, a request is started, and the code runs without blocking the result.
This is vital in JavaScript because it is a very natural fit for the user interface code and very efficient performance-wise on the server end.
The WeakMap object is basically a collection of key or value pairs where the keys are weakly referenced. It provides a way for extending objects from the outside without meddling into the garbage collection.
Here are some use cases of Weakmap objects:
- Maintaining private data about a specific object while only giving access to people having a reference to the Map.
- Safekeeping of data related to the library objects without making any changes to them or incurring any overhead.
- Keeping of data related to the host objects such as DOM nodes in the browser.
The main difference between these two is when you are using inheritance. It is much more complicated using inheritance in ES5, while the ES6 version is simple and easy to remember.
ES6 Class:
class Person {
constructor(name) {
this.name = name;
}
}
ES5 function constructor:
function Person(name) {
this.name = name;
}
In JavaScript, generators are those functions which can be exited and re-entered later on. Their variable bindings shall be saved across the re-entrances. They are written using the function* syntax.
The Generator function should be used when:
- You can choose to jump out of a function and let the outer code determine when to jump back in the function.
- The control of the asynchronous call can be executed outside of your code.
JavaScript is a high-level, multi-paradigm programming language which conforms to the ECMAScript specification. Read our list of advanced javascript interview questions to get a deep understanding of this language.
One of the drawbacks of creating a true private method in JavaScript is that they are highly memory consuming. A new copy for each method is created for every instance.
Example:
var Employee = function (name, company, salary) {
this.name = name || "";
this.company = company || "";
this.salary = salary || 5000;
var increaseSlary = function () {
this.salary = this.salary + 1000;
};
this.dispalyIncreasedSalary = function() {
increaseSalary();
console.log(this.salary);
};
};
var emp1 = new Employee("Amar","Mars",3000);
Here, while creating each variable, each instance makes a new copy of emp1, emp2, and emp3 in IncreaseSalary. Thus, making it inefficient for your server end.
Top 10 Advanced JavaScript Interview Questions
Here you will find the list of Top Advanced JavaScript Interview Questions, which were written under the supervision of industry experts. These are the most common questions and usually asked in every interview for the role of the JavaScript Developers.
- What is prototype?
- What is Callback hell?
- What is callback?
- What are the difference between arrow functions & normal functions?
- What is JavaScript Hoisting?
- What are the difference between let, var & const?
- What is Promises?
- What is the difference between Map & filter function?
- How to remove duplicate values in array?
- What are Closure?
- What is rest & spread operators?
- What are the data types used in JavaScript?