Advanced JavaScript Interview Questions
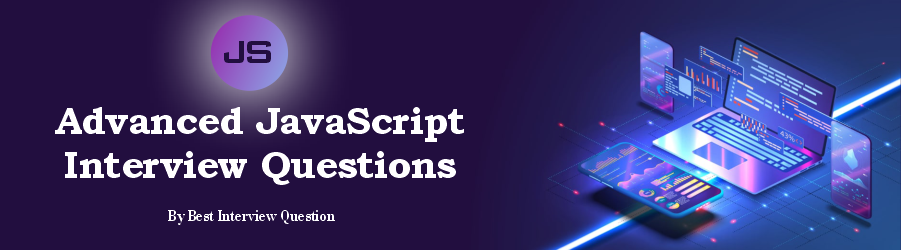
Over the years, JavaScript has become one of the most influential programming languages in the software industry. Due to this, there are a number of job applications and applicants in this industry. Due to this, we decided to come up with the actual Advanced JavaScript Interview Questions, questions that actually matter while you create codes for your company. Have a read on these questions to cement your knowledge in JavaScript and get that dream job. But, over time, we have found out that many developers lack a basic understanding of JavaScript such as working on objects and working on obsolete patterns like deeply layered inheritance hierarchies.
What's in it for me?
We have created this section for your convenience from where you can navigate to all the sections of the article. All you need to just click or tap on the desired topic or the section, and it will land you there.
JavaScript Coding Interview Questions
A function that can access its parent scope, even after the parent function has closed is called JavaScript closure.
const parentMethod = () => {
const title = 'Advanced JavaScript Questions';
const innerMethod = () => {
console.log(title);
}
innerMethod();
}
parentMethod();
In other words, a closure gives you access to an outer function's scope from an inner function.
const items = [40, 10, 11, 15, 25, 21, 5];
Find max value
console.log(Math.max(...items)); // 40
Find min value
console.log(Math.min(...items)); // 5
let x = null;
console.log(Math.round(x));
0
const displayTable = (number) => {
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const countingArray = numbers.map((item) => {
console.log(item*number);
});
}
displayTable(2);
JavaScript is a dynamic language and at any time we can add new properties to an object and can be shared among all the instances. Hence to add new properties and implement inheritance prototypes are used.
Example
function Candidate() {
this.name = 'Arun';
this.role = 'QA';
}
var candidateObj1 = new Candidate();
candidateObj1.salary = 10000;
console.log(candidateObj1.salary); // 10000
var candidateObj2 = new Candidate();
console.log(candidateObj2.salary); // undefined
The following are the different types of data types used in JavaScript.
- Numbers: Can represent both floating-point and integer numbers. Example: 5, 6.5, 7 etc.
- String: A sequence of characters is called a string. Strings can be enclosed in JavaScript within single or double quotes. Example: "Advanced JavaScript Questions" etc.
- Boolean: This represents a logical entity. It can have either true or false values.
- Null: This type only has one value: null
- Undefined: A variable whose value has yet to be assigned is undefined.
- Symbol: It is not a primitive data type that can be used to represent literal forms. It is a built-in object whose constructor returns a unique symbol.
- bigint: This is the bigint type that represents whole numbers larger than 253-1. You must add the letter n to the end of the number in order to form a literal bigint number.
- Object: This is the most crucial data-type. It forms the foundation for modern JavaScript. These data types will be discussed in more detail in future articles.
Note: We have a list of all interview questions related to ES6. These are really helpful in cracking your interviews.
const displayText = () => {
console.log("A");
setTimeout(() => {
console.log("B");
}, 0)
console.log("C");
}
displayText();
A
C
B
const res = [];
res.x = 5;
res.x = 10;
console.log(res);
[ x: 10 ]
const list = [1, 2, 3, 4, 5, 6];
const list2 = [5, 6, 7, 8, 9, 10];
console.log([...list, ...list2]);
[ 1, 2, 3, 4, 5, 6, 5, 6, 7, 8, 9, 10 ]
const list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const result = list.find((item) => {
return item > 3;
})
console.log(result);
4
Top 10 Advanced JavaScript Interview Questions
Here you will find the list of Top Advanced JavaScript Interview Questions, which were written under the supervision of industry experts. These are the most common questions and usually asked in every interview for the role of the JavaScript Developers.
- What is prototype?
- What is Callback hell?
- What is callback?
- What are the difference between arrow functions & normal functions?
- What is JavaScript Hoisting?
- What are the difference between let, var & const?
- What is Promises?
- What is the difference between Map & filter function?
- How to remove duplicate values in array?
- What are Closure?
- What is rest & spread operators?
- What are the data types used in JavaScript?